Hi,
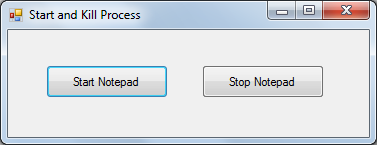
Let's start;
Steps
1. Open Visual Studio and Create a New Project -> Windows Form Application with
Name StartKillProcess.
2. Now add 2 buttons to your empty form and design it as shown in the below screenshot.
Also change the button names to;
Name Text
btnStart Start Notepad
btnStop Stop Notepad
3. Now double click on Start Notepad button and copy the below code;
//to open notepad, for calculator give "calc"
System.Diagnostics.Process.Start("Notepad");
4. Also double click on Stop Notepad button and copy the below code;
Process[] processes = null;
processes = Process.GetProcessesByName("Notepad");
foreach (Process process in processes)
{
process.Kill();
}
5. The full source code is shown below;
Form1.cs
using System;
using System.Diagnostics;
using System.Windows.Forms;
namespace StartKillProcess
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnStart_Click(object sender, EventArgs e)
{
System.Diagnostics.Process.Start("Notepad");
}
private void btnStop_Click(object sender, EventArgs e)
{
Process[] processes = null;
processes = Process.GetProcessesByName("Notepad");
foreach (Process process in processes)
{
process.Kill();
}
}
}
}
6. Now Run the project and click on Start Notepad button to open Notepad and click on
Stop Notepad button to close the Notepad.
Output
Conclusion
- You have learned to Start and Kill a Process in C#. If you have any doubts or errors please comment it.
See Also
Exactly what I was searching for..!
ReplyDelete